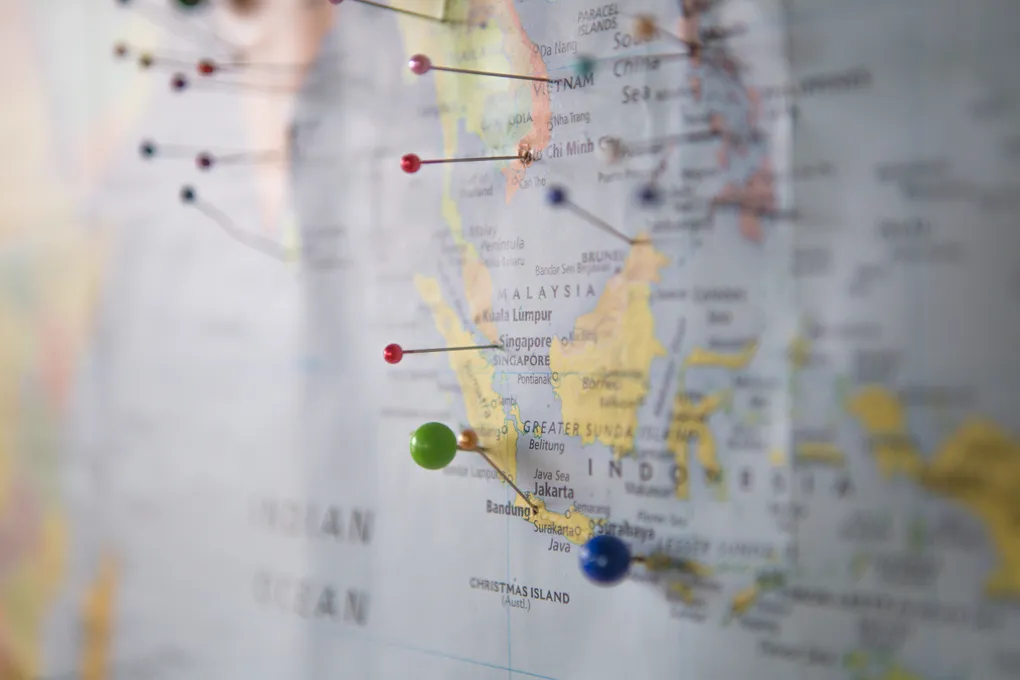
Javascript's map function in Go
Posted
If you have written any javascript, you have probably used the array function map. Here is a short example:
const dogs = [
{
name: "One",
breed: "Retriever",
},
{
name: "Two",
breed: "Beagle",
},
];
const dogNames = dogs.map((dog) => {
return dog.name;
});
console.log(dogNames);
// output: ["One", "Two"]
This is pretty handy if you have an array and you wanna trim it down or extract some of the data into a new array.
Now let’s see how we can do the same thing in go:
dogs := []dog{
{
Name: "One",
Breed: "Retriever",
},
{
Name: "Two",
Breed: "Beagle",
},
}
dogNames := func(dogs []dog) []string {
var dogNames []string
for _, d := range dogs {
dogNames = append(dogNames, d.Name)
}
return dogNames
}(dogs)
fmt.Println(dogNames)
// output: [One Two]
There you have it, a simple and fast way to create a new array based on another one with just the data you need.
Thank you for reading.