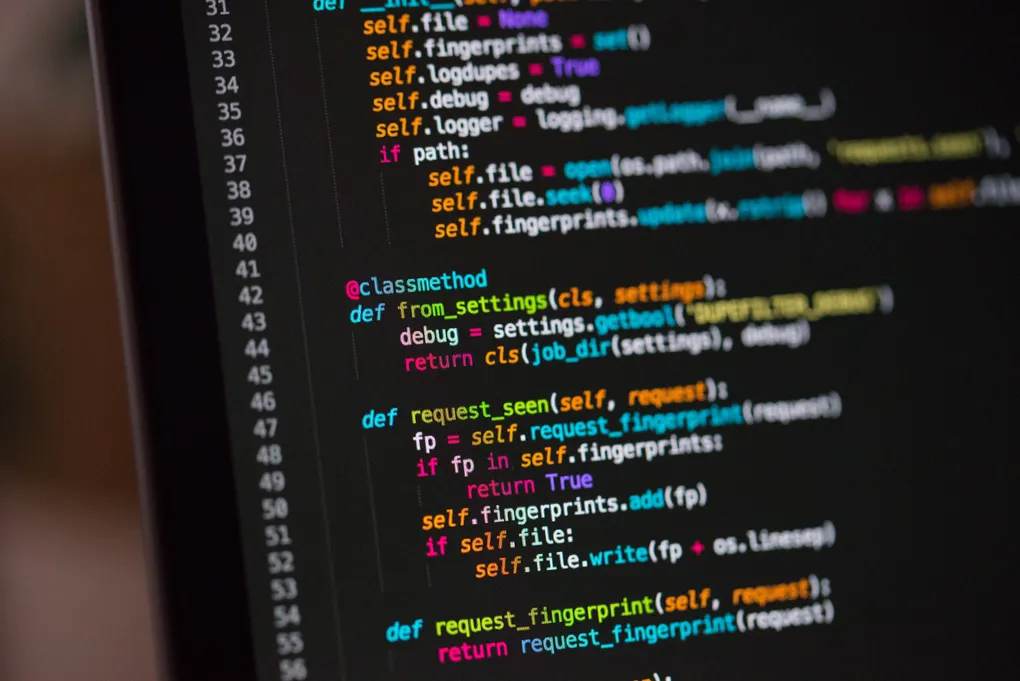
Get started with go and postgres in a giffy
Posted
I wanna show you how you get quickly started with postgres in your go application.
First we create our docker-compose.yml
file:
version: '2'
services:
db:
image: postgres
restart: unless-stopped
ports:
- "5432:5432"
environment:
LC_ALL: C.UTF-8
POSTGRES_USER: root
POSTGRES_PASSWORD: root
POSTGRES_DB: db_example
Then we start our postgres database with docker-compose up -d
.
The -d
flag make docker-compose
run the container in the background.
Now we write a simple go program to test our connection to the newly created database. In this example I use the pq postgres driver.
package main
import (
"database/sql"
"log"
_ "github.com/lib/pq"
)
func main() {
// make sure these credentials match
// those that you put in the docker-compose file.
connectionString := "user=root password='root' dbname=db_example sslmode=disable"
db, err := sql.Open("postgres", connectionString)
if err != nil {
log.Println("sql.Open", err)
return
}
// make sure we are connected to the database.
if err := db.Ping(); err != nil {
log.Println("db.Ping", err)
}
log.Println("Success!")
}
Run the program with go run main.go
.
Now if you see Success!
in the terminal everything worked, and you are ready to continue using postgres in your application.
Thank you for reading.