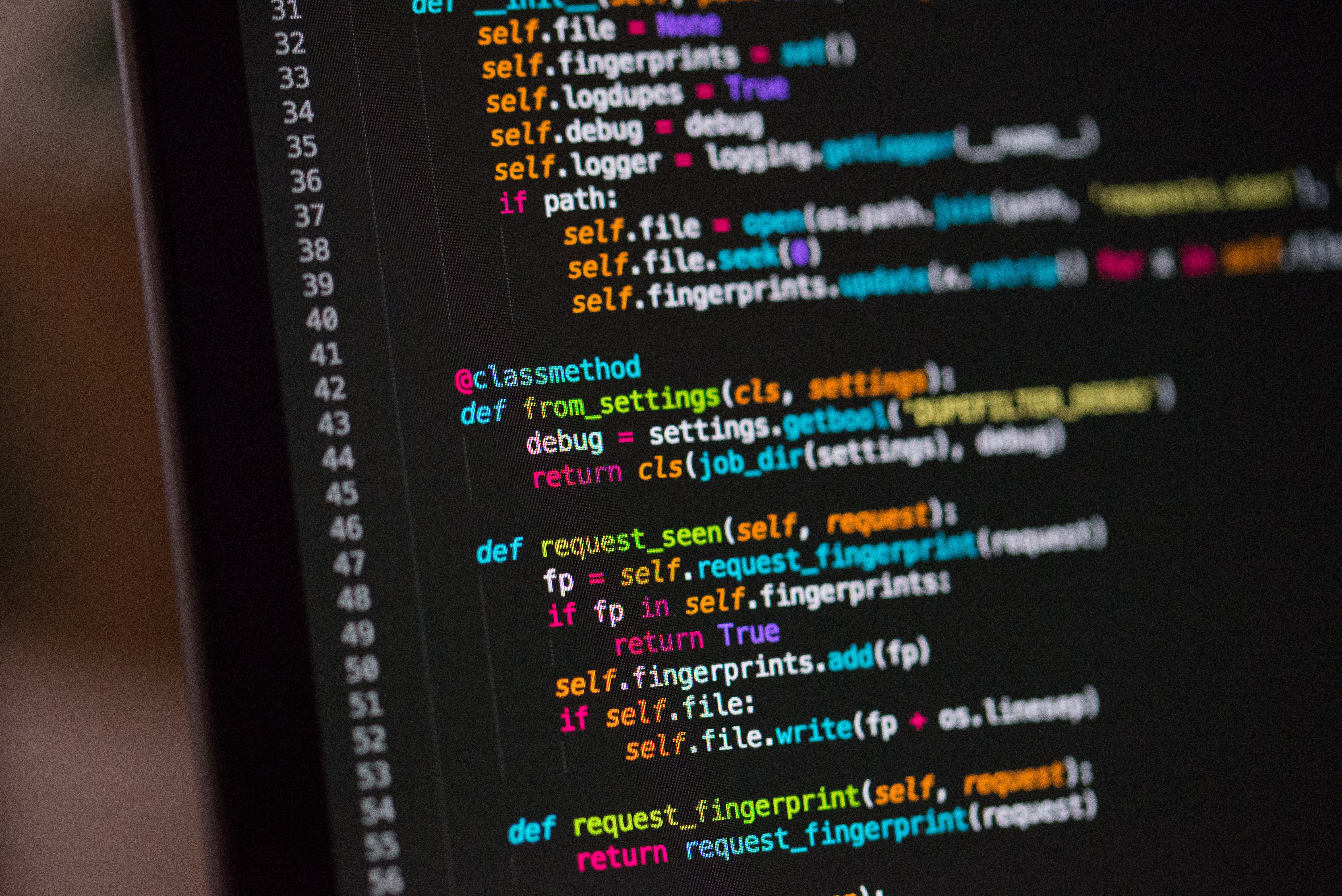
Cancel database query with context
A simple example on how cancel a query with context.
An short example of how we can use the context package to cancel an query request. We are gonna use the WithTimeout function to do this.
First a simple webserver:
func main() {
port := ":8000"
// query route
http.HandleFunc("/query", query)
log.Println("Listening on " + port)
log.Fatal(http.ListenAndServe(port, nil))
}
The database handler:
func query(w http.ResponseWriter, r *http.Request) {
// We create a new context with an given timeout at 1 second
ctx, cancel := context.WithTimeout(r.Context(), time.Second*1)
defer cancel()
// simulating an database query
time.Sleep(time.Second * 2)
// If the timeout is exceeded ctx.Done() will be triggered
select {
case <-ctx.Done():
w.Write([]byte(ctx.Err()))
default:
w.Write([]byte(`timeout not exceeded`))
}
}
Thanks for reading